Whenever we get empty response from database or internal API call, we should return a structured, meaningful response should return to the client, even when no data is available.
Let’s understand problem
Consider below code.
[ApiController]
[Route("[controller]")]
public class NullResponseController : ControllerBase
{
private readonly ILogger<NullResponseController> _logger;
private readonly Dictionary<int, string> _usersDetails = new Dictionary<int, string>
{
{ 1, "Devesh omar" },
{ 2, "Ram Kumar" }
};
public IActionResult GetUser(int id)
{
if (_usersDetails.TryGetValue(id, out var user))
{
return Ok(user); // Return user details
}
// Returns null response
return Ok();
}
public NullResponseController(ILogger<NullResponseController> logger)
{
_logger = logger;
}
}
a. Unintentional Mistakes:
In this code (GetUser API)we are sending blank or empty data to client when data not found in database. These code cause confusion to UI developer, and he will never understand if there are some problem in API side or he is getting blank data accidently.
Let’s Execute below code or endpoint and see what response we get if we send empty or just return ok();
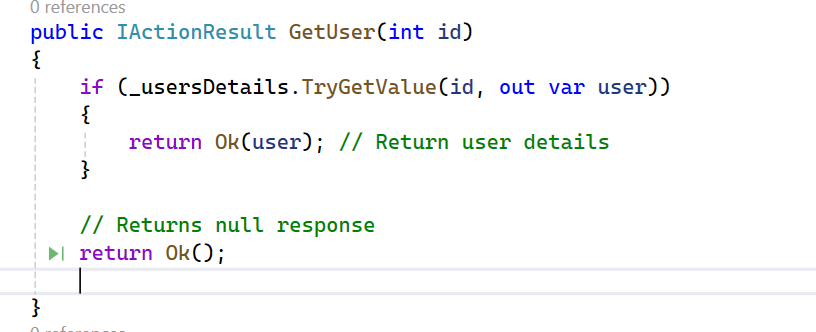
When we executed below endpoint, we can see we are getting blank response and response body is blank.
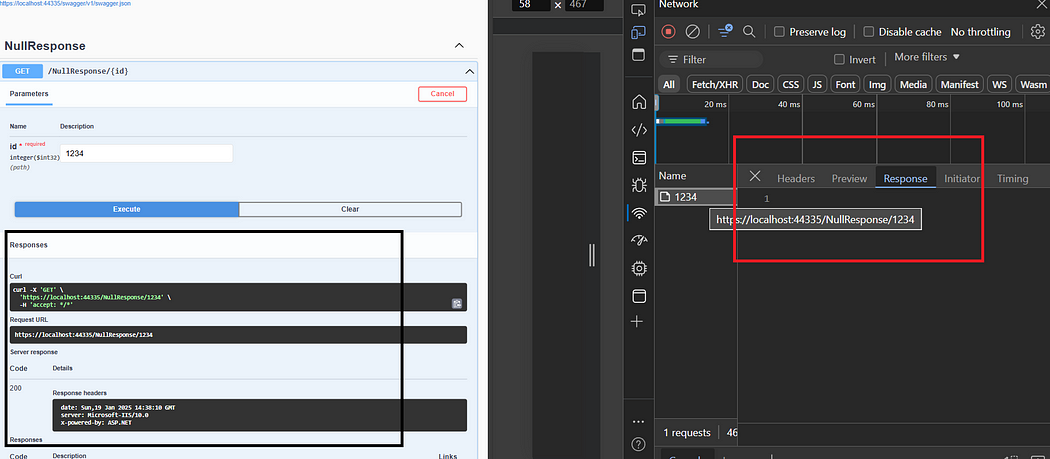
b. Client-Side issues.
Many clients (e.g., JavaScript-based apps) do not handle null
or empty responses gracefully, potentially leading to runtime errors like:
Cannot read property of null
Example: A client expecting a JSON object receives an empty response and crashes because JSON.parse()
fails.
c. Unexpected HTTP Status Codes
if api those returning empty response and still sending HTTP status 200 , that can mislead clients into thinking the request was successful when there was no data to process.
In such cases we should respond with proper status codes like 204 No Content should
Solution: Custom NullResponseMiddleWare
To handle above scenario we can create NullResponseMiddleware which will see if response is null then it will send default message to client
Let’s create custom Middleware
public class NullResponseHandlingMiddleware
{
private readonly RequestDelegate _next;
public NullResponseHandlingMiddleware(RequestDelegate next)
{
_next = next;
}
public async Task InvokeAsync(HttpContext context)
{
// Create a memory stream to intercept the response
using var memoryStream = new MemoryStream();
var originalBodyStream = context.Response.Body;
context.Response.Body = memoryStream;
// Proceed with the pipeline
await _next(context);
// Reset the stream position to read the response
memoryStream.Seek(0, SeekOrigin.Begin);
var responseBody = await new StreamReader(memoryStream).ReadToEndAsync();
// If the response body is empty, replace it
if (string.IsNullOrWhiteSpace(responseBody) && context.Response.StatusCode == 200 || context.Response.StatusCode == 204)
{
context.Response.Body = originalBodyStream; // Reset the original stream
context.Response.ContentType = "application/json";
await context.Response.WriteAsync("{\"message\": \"No content available for this API.\"}");
}
else
{
// Copy the original response body back to the stream
memoryStream.Seek(0, SeekOrigin.Begin);
await memoryStream.CopyToAsync(originalBodyStream);
}
}
}
Implementation details
a. We are creating custom middleware with name NullResponseHandlingMiddleware
b. Inside Invoke Method we will read response body and will see if response if blank or null , if response if blank then we will send Default message (line number 32 in below code), else respond with original data.
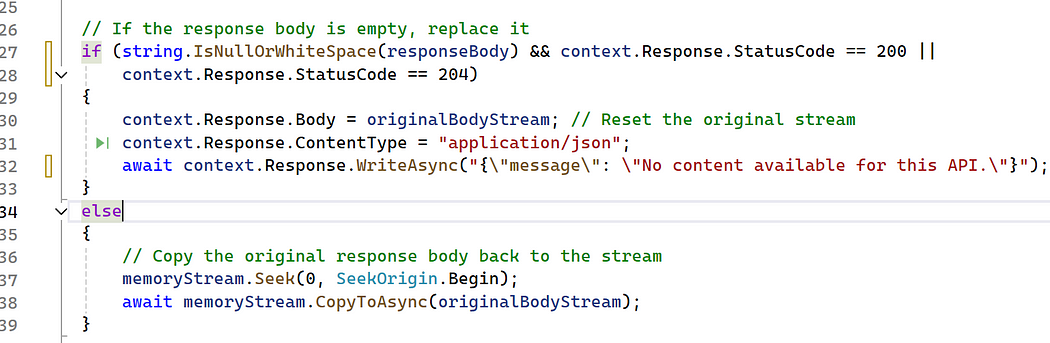
Register middleware at startup.cs
var builder = WebApplication.CreateBuilder(args);
// Add services to the container.
builder.Services.AddControllers();
// Learn more about configuring Swagger/OpenAPI at https://aka.ms/aspnetcore/swashbuckle
builder.Services.AddEndpointsApiExplorer();
builder.Services.AddSwaggerGen();
var app = builder.Build();
// Configure the HTTP request pipeline.
if (app.Environment.IsDevelopment())
{
app.UseSwagger();
app.UseSwaggerUI();
}
app.UseMiddleware<NullResponseHandlingMiddleware>();
Code execution
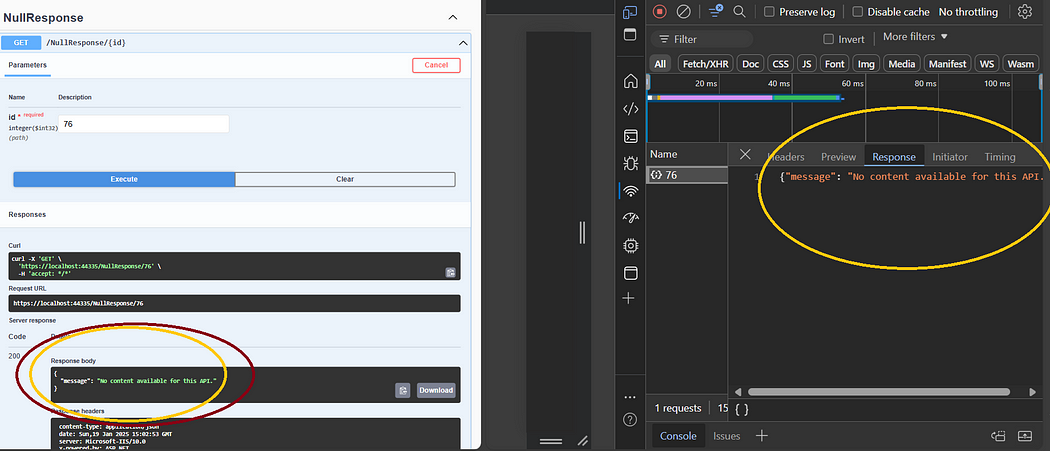
After code execution we can see if we are sending blank data then we will be getting default message.
This Solution work well in scenario when we have 1000’s of API in application and we want to fix all end point in applications with this centralize solution.
Thanks.
Happy Coding and Happy learning
Comments
Post a Comment