Situation will become unmanageable if we have lot of if else condition in our code, Lets understand problem and then we will see it’s Solution.
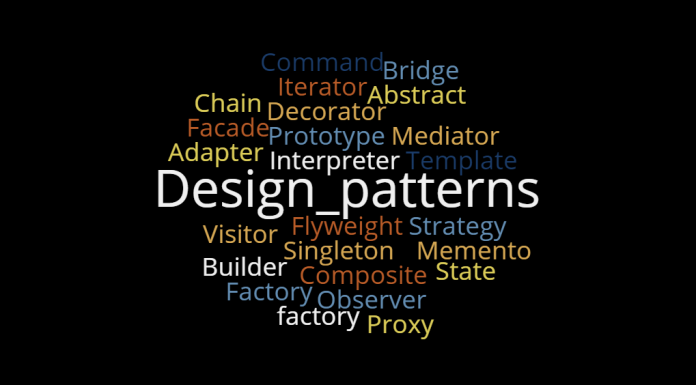
Problem with Multiple If Conditions
Using multiple if-else conditions to handle different cases can lead to code that is hard to read, maintain, and extend. Here’s a simple example using multiple if conditions in a leave management system.
We will implement below Business Logic for Leave management system
a. IF Leave < = 3, then Team Lead will approve
b. IF Leave < =5, then Project Manager will approve
c. IF Leave > 5, then HR Manager will approve
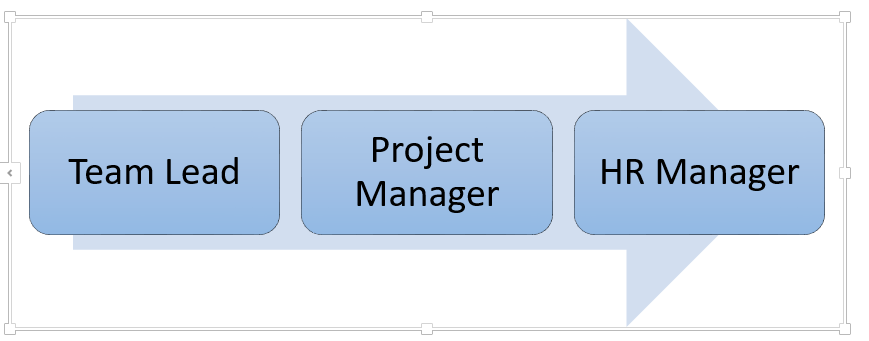
Request first go to team lead to check if Request can be handled by Team lead else it will go to Project Manager else request will go to HR manager
Example with Multiple If Conditions
public class LeaveRequest
{
public string EmployeeName { get; set; }
public int NumberOfDays { get; set; }
public string Reason { get; set; }
}
public class LeaveApprovalService
{
public void ApproveLeave(LeaveRequest request)
{
if (request.NumberOfDays <= 3)
{
Console.WriteLine($"Leave request by {request.EmployeeName} for {request.NumberOfDays} days approved by Team Lead.");
}
else if (request.NumberOfDays <= 5)
{
Console.WriteLine($"Leave request by {request.EmployeeName} for {request.NumberOfDays} days approved by Project Manager.");
}
else if (request.NumberOfDays > 5)
{
Console.WriteLine($"Leave request by {request.EmployeeName} for {request.NumberOfDays} days approved by HR Manager.");
}
else
{
Console.WriteLine("Leave request could not be processed.");
}
}
}
Problems in above code
- Maintainability: The method becomes harder to maintain as the logic grows.
- Single Responsibility Principle (SRP): The method does too much by handling all approval levels, violating the single responsibility principle.
- Testing : Each time we add new condition we need to test end to end to make sure nothing is breaking because we are making changes into existing class because we are breaking SRP.
Solution with Chain of Responsibility Pattern
Chain of Responsibility Pattern or Chain of Responsibility Method is a Behavioral Design Pattern, which allows an object to send a request to other objects without knowing who is going to handle it.
This pattern encourages loose coupling between sender and receiver, providing freedom in handling the request.
Implementation Details
Centralize handler
1. This centralizes handler provides a default implementation for handling the request, which includes logic to either process the request or pass it to the next handler in the chain if it cannot handle it.
2. This centralizes handler maintains a reference to the next handler in the chain. This allows each handler to pass the request along the chain if it cannot handle the request.
3. By defining how requests are passed from one handler to the next, the abstract handler encapsulates this logic, reducing duplication and simplifying the concrete handlers.
Code Implementation
Leave Request class
public class LeaveRequest
{
public string EmployeeName { get; set; }
public int NumberOfDays { get; set; }
public string Reason { get; set; }
}
This class contains details of employee who is takig leave and why and how many days
2. Centralize Handler Creation
public abstract class LeaveHandler
{
protected LeaveHandler NextHandler;
public void SetNextHandler(LeaveHandler nextHandler)
{
NextHandler = nextHandler;
}
public void HandleRequest(LeaveRequest request)
{
if (CanHandle(request.NumberOfDays))
{
ProcessRequest(request);
}
else if (NextHandler != null)
{
NextHandler.HandleRequest(request);
}
else
{
Console.WriteLine("Leave request could not be processed.");
}
}
protected abstract bool CanHandle(int numberOfDays);
protected abstract void ProcessRequest(LeaveRequest request);
}
3. Concrete Handlers
Here we will create separate classes for Team lead, HR manager, project manager as below.
public class TeamLead : LeaveHandler
{
protected override bool CanHandle(int numberOfDays) => numberOfDays <= 3;
protected override void ProcessRequest(LeaveRequest request)
{
Console.WriteLine($"Leave request by {request.EmployeeName} for {request.NumberOfDays} days approved by Team Lead.");
}
}
public class ProjectManager : LeaveHandler
{
protected override bool CanHandle(int numberOfDays) => numberOfDays <= 5;
protected override void ProcessRequest(LeaveRequest request)
{
Console.WriteLine($"Leave request by {request.EmployeeName} for {request.NumberOfDays} days approved by Project Manager.");
}
}
public class HRManager : LeaveHandler
{
protected override bool CanHandle(int numberOfDays) => numberOfDays > 5;
protected override void ProcessRequest(LeaveRequest request)
{
Console.WriteLine($"Leave request by {request.EmployeeName} for {request.NumberOfDays} days approved by HR Manager.");
}
}
Client code for testing
using Chainofresponbility;
Console.WriteLine("Welcome to .Net Labs !");
Console.WriteLine(".........................");
Console.WriteLine("Example of Chain of Responsibility !");
Console.WriteLine(".........................");
Console.WriteLine(".........................");
// Create handlers
var teamLead = new TeamLead();
var projectManager = new ProjectManager();
var hrManager = new HRManager();
// Set up the chain
teamLead.SetNextHandler(projectManager);
projectManager.SetNextHandler(hrManager);
// Create a leave request
var leaveRequest = new LeaveRequest
{
EmployeeName = "Devesh",
NumberOfDays = 4,
Reason = "Sick Leave"
};
// Process the request
teamLead.HandleRequest(leaveRequest);
// Another leave request
var anotherLeaveRequest = new LeaveRequest
{
EmployeeName = "Sanjay Singh",
NumberOfDays = 6,
Reason = "Vacation"
};
// Process the request
teamLead.HandleRequest(anotherLeaveRequest);
Console.ReadKey();
Code explanation
a. Set up chain -> Request will go to team lead and next handler will be project manager and project manager have next handler as Hr manager,
// Set up the chain
teamLead.SetNextHandler(projectManager);
projectManager.SetNextHandler(hrManager);
b. Processing Request
// Create a leave request
var leaveRequest = new LeaveRequest
{
EmployeeName = "Devesh",
NumberOfDays = 4,
Reason = "Sick Leave"
};
// Process the request
teamLead.HandleRequest(leaveRequest);
Output
We can see Leave request for devesh handled by Project manager because it have leave count =4
Also we can see Leave request for Sanjay handled by HR manager because it have leave count =6
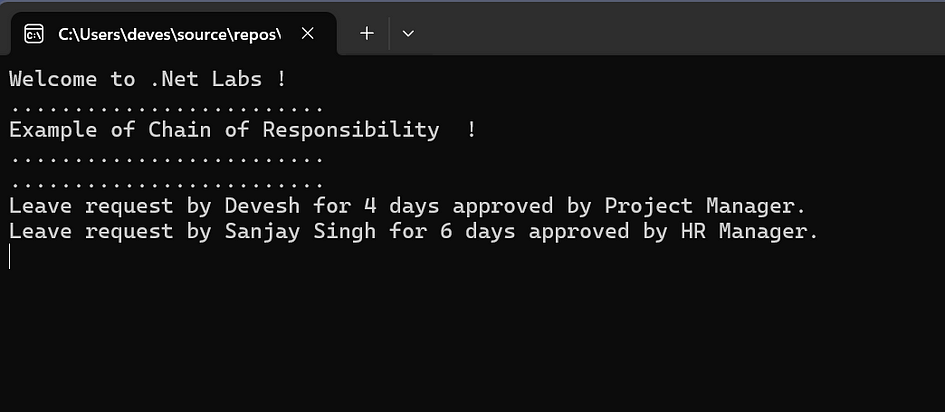
Thanks
Happy Coding
Comments
Post a Comment