This pattern can be ideal approach to create complex objects
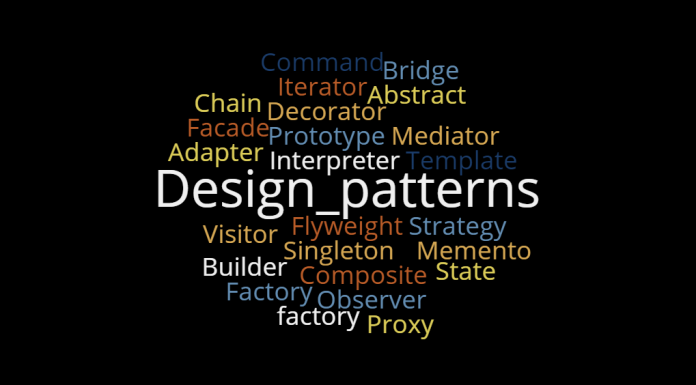
The Builder pattern is a creational design pattern that is used to construct complex objects step by step. It allows you to create different representations of an object using the same construction process.
Let’s first understand the problems that exist without using this pattern.
- Complex Constructor with Many Parameters
When creating objects that require many parameters, constructors can become lengthy and hard to read.
public class House
{
public House(int walls, int doors, int windows, bool hasGarage, bool hasSwimmingPool, bool hasStatues, bool hasGarden)
{
// Initialization
}
}
There are many problems if constructor have too many parameters
i) Each time we need to pass n numbers of parameters at time of object creations
ii) we need to update constructor if we need to pass one more parameter so such constructor difficult to manage
2. Constructor Overload Explosion
Sometimes we need to create overloaded constructor based on parameters, so managing multiple constructors again have some headache.
To handle different combinations of parameters, multiple constructor overloads are often created, leading to code duplication and increased maintenance.
public House(int walls, int doors) { ... }
public House(int walls, int doors, int windows) { ... }
public House(int walls, int doors, int windows, bool hasGarage) { ... }
3. Readability and Maintainability Issues:
Code that creates instances with many parameters becomes hard to read and maintain.
var house = new House(4, 2, 6, true, false, true, true);
Introduction of Builder pattern to fix those issues
The Builder pattern breaks down the construction of a complex object into simpler steps. Each step builds a part of the product, and the final step assembles the product.
public class HouseBuilder
{
private int _walls;
private int _doors;
private int _windows;
private bool _hasGarage;
private bool _hasSwimmingPool;
private bool _hasStatues;
private bool _hasGarden;
public HouseBuilder SetWalls(int walls) { _walls = walls; return this; }
public HouseBuilder SetDoors(int doors) { _doors = doors; return this; }
public HouseBuilder SetWindows(int windows) { _windows = windows; return this; }
public HouseBuilder SetGarage(bool hasGarage) { _hasGarage = hasGarage; return this; }
public HouseBuilder SetSwimmingPool(bool hasSwimmingPool) { _hasSwimmingPool = hasSwimmingPool; return this; }
public HouseBuilder SetStatues(bool hasStatues) { _hasStatues = hasStatues; return this; }
public HouseBuilder SetGarden(bool hasGarden) { _hasGarden = hasGarden; return this; }
public House Build()
{
return new House(_walls, _doors, _windows, _hasGarage, _hasSwimmingPool, _hasStatues, _hasGarden);
}
}
Object creations
var house = new HouseBuilder()
.SetWalls(4)
.SetDoors(2)
.SetWindows(6)
.SetGarage(true)
.SetSwimmingPool(false)
.SetStatues(true)
.SetGarden(true)
.Build();
Benefits
a. Creation of object with this pattern is very much readable, here we can understand what we are passing
b. We can control default values and validation from set methods
public HouseBuilder SetDoors(int doors) {
if (_door == 0) {
_doors = 4 // default value
} else {
_door = door;
}
return this;
}
c. We can make DB calls if required from setMethods
public HouseBuilder SetDoors(int doors) {
if (_door == 0) {
// Make a DB call to get default value
} else {
_door = door;
}
return this;
}
d. Object creation with Less Parameters
var house = new HouseBuilder()
.SetWalls(4)
.SetDoors(2)
.SetWindows(6)
.Build();
we have removed all bool parameters from above in this case we can set up default values if something we are not passing any parameters.
e. By using the Builder pattern, the object can be made immutable since the builder itself holds the state until the Build method is called. we can called this as this pattern Encourages Immutability
Thanks, Happy Coding and Learnings
#designpattern
#dotnet
#dotnetcore
#Design
Comments
Post a Comment